The Lumify Contact Form SDK provides advanced functionality to customize styling and functionality of your contact form widgets. This SDK replaces the need to use the default <iframe>
method. The SDK provides JS methods to manage data collection, validation error display, form submission, and success / error messaging.
Installation
Include the Contact Form SDK in your website. You can either reference the Lumify SDK using our CDN:
<script src="https://lumify.app/sdk/contact-form-sdk.js" type="text/javascript"></script>
Or you can download the SDK from here and include it on your site manually.
Initialization
To initialize the SDK, create a new instance of the LumifyContactFormSdk
class by passing the form element, your contact form API endpoint, and an optional configuration object.
async function handleContactForm(event) {
event.preventDefault();
/**
* Update these fields
*/
const tenantUrl = 'lumosevents.lumify.app'; // your tenant subdomain
const contactFormId = '9b6069b6-7bf7-4d72-b3d8-8eab3f0f6f44'; // the contact form id
/**
* Advanced users for any other updates...
*/
const lumifySdk = new LumifyContactFormSdk(
event.target,
`https://${tenantUrl}/api/widget/contact-form/${contactFormId}`
);
// ...handle form submission
}
Additional Configuration (optional)
formMessageClass
: The class name for the form message element. Default is: 'lumify-form-message'. This allows you to display success and error messages on your contact form. Do not include the '.' (dot) for your custom class names.fieldErrorClass
: The class name for the field error elements. Default is: 'lumify-field-error'. This allows you to display error messages under any fields that fail form validation. Do not include the '.' (dot) for your custom class names.
Required Form Fields
When using the SDK, ensure you are matching the custom HTML contact form fields to the form fields included on your Lumify contact form. The fields should be a 1-to-1 match for at least any 'required' fields. Optional fields can be ignored in your custom HTML.
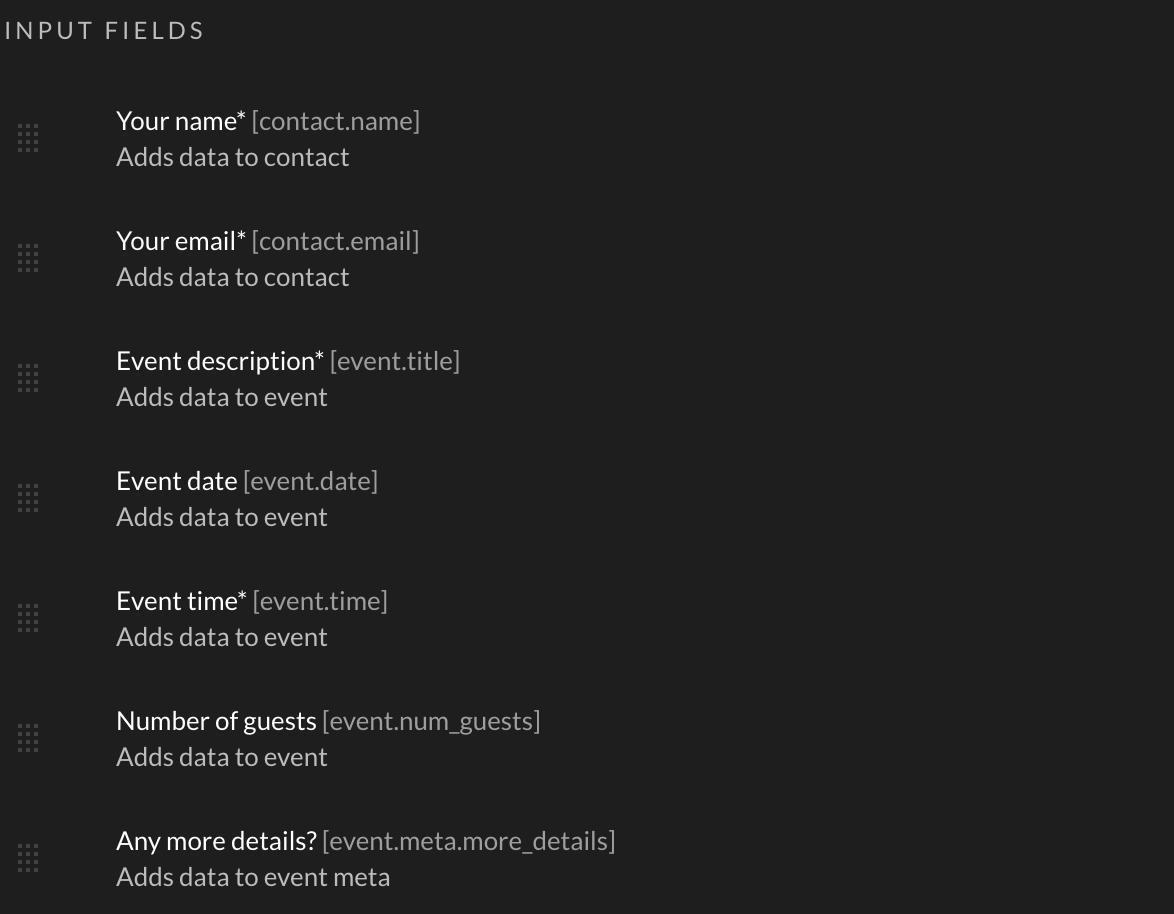
Form Validation
In order to provide helpful validate messages, you should use the showFieldErrors
. For this to show relevant validation errors, you must include an HTML element with the .lumify-field-error
class near each form field (see example below).
Available Methods
Method | Description | Parameters |
submitForm() | Submits the contact form to the specified tenant URL. | |
showFormMessage(message, state) | Displays a message on the form. | message (string): The message to display for the form. state (enum<success|error>): The state of the form message (defaults to 'success') |
clearFormMessage() | Clears any form messages. | |
showFieldErrors(errors) | Displays validation errors under each field. | errors (object): Key-value pair of errors. The key must be associated with a named field. |
clearFieldErrors() | Clears all field errors. | |
resetForm() | Resets form values, messages, and errors. | |
errorData | This is not a method, but this class parameter can be useful to dsplay validation errors. |
Complete Example
Most use cases can use the following example as a starting point:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Lumify Contact Form</title>
<style>
body {
margin: 75px;
}
.lumify-form-message {
font-size: 0.9em;
min-height: 0;
}
.lumify-form-message-success {
color: green;
}
.lumify-form-message-error {
color: red;
}
.lumify-field-error{
color: red;
font-size: 0.9em;
min-height: 0;
}
</style>
</head>
<body>
<script src="https://lumify.app/sdk/contact-form-sdk.js" type="text/javascript"></script>
<script type="text/javascript">
async function handleContactForm(event) {
event.preventDefault();
/**
* Update these fields
*/
const tenantUrl = 'lumosevents.lumify.app';
const contactFormId = '9b6069b6-7bf7-4d72-b3d8-8eab3f0f6f44';
/**
* Advanced users for any other updates...
*/
const lumifySdk = new LumifyContactFormSdk(
event.target,
`https://${tenantUrl}/api/widget/contact-form/${contactFormId}`
);
try {
await lumifySdk.submitForm(endpoint);
lumifySdk.resetForm();
lumifySdk.showFormMessage('Got your message! We\'ll be in touch soon! Thanks!', 'success');
} catch (error) {
lumifySdk.showFormMessage(error.message, 'error');
// lumifySdk.showFormMessage(lumifySdk.errorData.message, 'error'); // another way to get validation errors
if (lumifySdk.errorData?.errors) {
lumifySdk.showFieldErrors(lumifySdk.errorData.errors);
}
}
}
</script>
<form id="lumify-contact-form" onsubmit="handleContactForm(event)">
<div class="lumify-form-message"></div>
<label for="contact.name">Name:</label>
<input type="text" id="contact.name" name="contact.name" required>
<div class="lumify-field-error lumify-field-error-contact-name"></div>
<br>
<label for="contact.email">Email:</label>
<input type="email" id="contact.email" name="contact.email" required>
<div class="lumify-field-error lumify-field-error-contact-email"></div>
<br>
<label for="event.title">Event Description:</label>
<input type="text" id="event.title" name="event.title" required>
<div class="lumify-field-error lumify-field-error-event-title"></div>
<br>
<label for="event.date">Event Date:</label>
<input type="date" id="event.date" name="event.date">
<div class="lumify-field-error lumify-field-error-event-date"></div>
<br>
<label for="event.time">Event Time:</label>
<input type="time" id="event.time" name="event.time">
<div class="lumify-field-error lumify-field-error-event-time"></div>
<br>
<label for="event.num_guests">Number of Guests:</label>
<input type="number" id="event.num_guests" name="event.num_guests">
<div class="lumify-field-error lumify-field-error-event-num_guests"></div>
<br>
<label for="event.meta.more_details">Anything else to share:</label>
<textarea id="event.meta.more_details" name="event.meta.more_details"></textarea>
<div class="lumify-field-error lumify-field-error-event-meta-more_details"></div>
<br>
<button type="submit">Submit</button>
</form>
</body>
</html>
Open Source
This SDK has been open sourced on GitHub. If you have issues or want to contribute, you can find the repository here.